Overexposure ~ Phase spikes
When studying complex surfaces, to be able to see the whole surface even the darkest areas, we need to increase the luminosity even if it means to overexpose some areas.
This choice introduces a noise on phase data. However this noise is known to be \(2nπ\). Therefore it is easy to detect, we just need to detect when the difference between two neighboring pixels is greater than \(2π\)
image_t DphaseSpike_ = image_t::Zero( nrows, ncols ); (1)
image_t pxU = image_t::Zero(nrows_, ncols_); // U = Up
image_t pxR = image_t::Zero(nrows_, ncols_); // R = Right
image_t pxD = image_t::Zero(nrows_, ncols_); // D = Down
image_t pxL = image_t::Zero(nrows_, ncols_); // L = Left
image_t pxUR = image_t::Zero(nrows_, ncols_);
image_t pxDR = image_t::Zero(nrows_, ncols_);
image_t pxDL = image_t::Zero(nrows_, ncols_);
image_t pxUL = image_t::Zero(nrows_, ncols_);(2)
pxU.block(1, 0, nrows_-1, ncols_) = (px.array().block(0, 0, nrows_-1, ncols_));
pxR.block(0, 0, nrows_, ncols_-1) = (px.array().block(0, 1, nrows_, ncols_-1));
pxD.block(0, 0, nrows_-1, ncols_) = (px.array().block(1, 0, nrows_-1, ncols_));
pxL.block(0, 1, nrows_, ncols_-1) = (px.array().block(0, 0, nrows_, ncols_-1));
pxUR.block(1, 0, nrows_-1, ncols_-1) = (px.array().block(0, 1, nrows_-1, ncols_-1));
pxDR.block(0, 0, nrows_-1, ncols_-1) = (px.array().block(1, 1, nrows_-1, ncols_-1));
pxDL.block(0, 1, nrows_-1, ncols_-1) = (px.array().block(1, 0, nrows_-1, ncols_-1));
pxUL.block(1, 1, nrows_-1, ncols_-1) = (px.array().block(0, 0, nrows_-1, ncols_-1));(3)
image_t dpxU = image_t::Zero(nrows_, ncols_);
image_t dpxR = image_t::Zero(nrows_, ncols_);
image_t dpxD = image_t::Zero(nrows_, ncols_);
image_t dpxL = image_t::Zero(nrows_, ncols_);
image_t dpxUR = image_t::Zero(nrows_, ncols_);
image_t dpxDR = image_t::Zero(nrows_, ncols_);
image_t dpxDL = image_t::Zero(nrows_, ncols_);
image_t dpxUL = image_t::Zero(nrows_, ncols_);
dpxU = (px.array()-pxU.array()).abs();
dpxR = (px.array()-pxR.array()).abs();
dpxD = (px.array()-pxD.array()).abs();
dpxL = (px.array()-pxL.array()).abs();
dpxUR = (px.array()-pxUR.array()).abs();
dpxDR = (px.array()-pxDR.array()).abs();
dpxDL = (px.array()-pxDL.array()).abs();
dpxUL = (px.array()-pxUL.array()).abs();(4)
image_t dpxMean = (dpxU.array() + dpxR.array() + dpxD.array() + dpxL.array()
+ dpxUR.array() + dpxDR.array() + dpxDL.array() + dpxUL.array()) / 8; (5)
image_t pyU = image_t::Zero(nrows_, ncols_);
image_t pyR = image_t::Zero(nrows_, ncols_);
image_t pyD = image_t::Zero(nrows_, ncols_);
image_t pyL = image_t::Zero(nrows_, ncols_);
image_t pyUR = image_t::Zero(nrows_, ncols_);
image_t pyDR = image_t::Zero(nrows_, ncols_);
image_t pyDL = image_t::Zero(nrows_, ncols_);
image_t pyUL = image_t::Zero(nrows_, ncols_);
pyU.block(1, 0, nrows_-1, ncols_) = (py.array().block(0, 0, nrows_-1, ncols_));
pyR.block(0, 0, nrows_, ncols_-1) = (py.array().block(0, 1, nrows_, ncols_-1));
pyD.block(0, 0, nrows_-1, ncols_) = (py.array().block(1, 0, nrows_-1, ncols_));
pyL.block(0, 1, nrows_, ncols_-1) = (py.array().block(0, 0, nrows_, ncols_-1));
pyUR.block(1, 0, nrows_-1, ncols_-1) = (py.array().block(0, 1, nrows_-1, ncols_-1));
pyDR.block(0, 0, nrows_-1, ncols_-1) = (py.array().block(1, 1, nrows_-1, ncols_-1));
pyDL.block(0, 1, nrows_-1, ncols_-1) = (py.array().block(1, 0, nrows_-1, ncols_-1));
pyUL.block(1, 1, nrows_-1, ncols_-1) = (py.array().block(0, 0, nrows_-1, ncols_-1));
image_t dpyU = image_t::Zero(nrows_, ncols_);
image_t dpyR = image_t::Zero(nrows_, ncols_);
image_t dpyD = image_t::Zero(nrows_, ncols_);
image_t dpyL = image_t::Zero(nrows_, ncols_);
image_t dpyUR = image_t::Zero(nrows_, ncols_);
image_t dpyDR = image_t::Zero(nrows_, ncols_);
image_t dpyDL = image_t::Zero(nrows_, ncols_);
image_t dpyUL = image_t::Zero(nrows_, ncols_);
dpyU = (py.array()-pyU.array()).abs();
dpyR = (py.array()-pyR.array()).abs();
dpyD = (py.array()-pyD.array()).abs();
dpyL = (py.array()-pyL.array()).abs();
dpyUR = (py.array()-pyUR.array()).abs();
dpyDR = (py.array()-pyDR.array()).abs();
dpyDL = (py.array()-pyDL.array()).abs();
dpyUL = (py.array()-pyUL.array()).abs();
image_t dpyMean = (dpyU.array() + dpyR.array() + dpyD.array() + dpyL.array()
+ dpyUR.array() + dpyDR.array() + dpyDL.array() + dpyUL.array()) / 8; (6)
DphaseSpike_ = (dpxMean.array() < M_PI && dpyMean.array() < M_PI).select(matrix_1, matrix_0); (7)
1 | Construction of a map with 0 everywhere |
2 | Construction of a 8 maps with 0 everywhere |
3 | Set those 8 maps as a copy of the original phasemap with an offset of one pixel in every possible direction |
4 | Compute the maps of phase differences between original map and its offsets |
5 | Compute the map of mean differences |
6 | Redo steps <1> to <5> for phases along y |
7 | Selection |
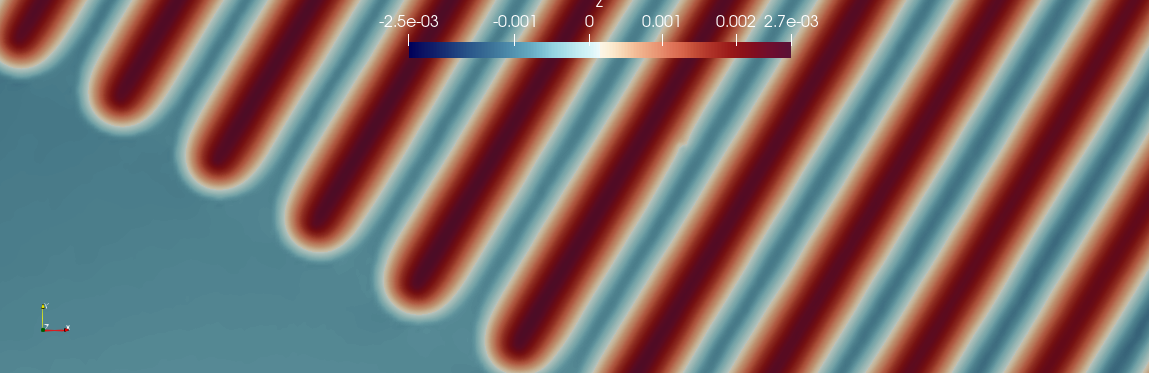
Figure 1. Suspect Area
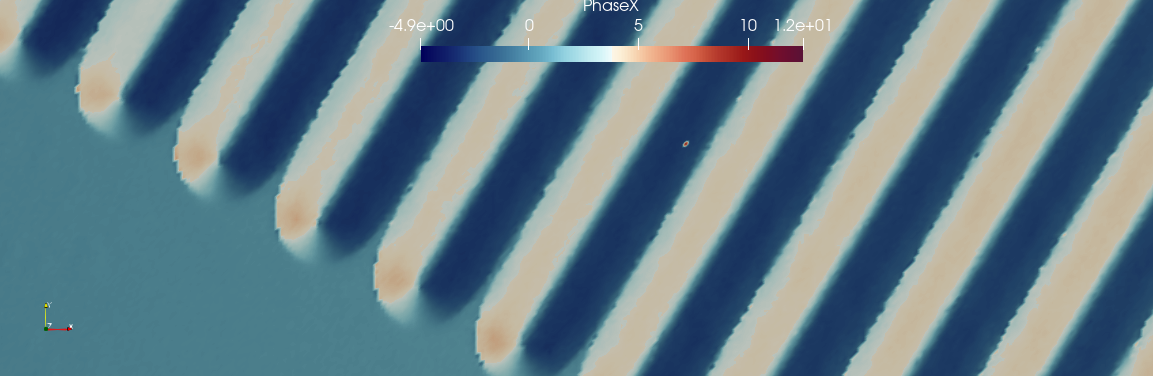
Figure 2. Phase map along X axis
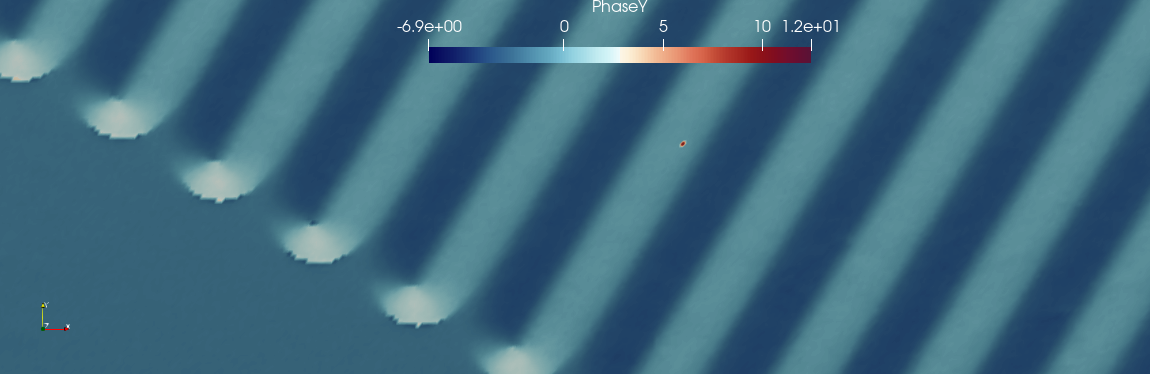
Figure 3. Phase map along Y axis
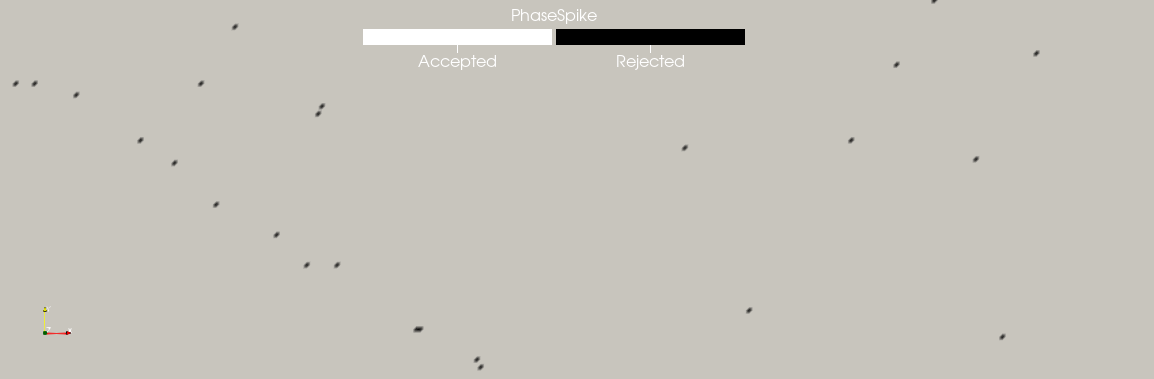
Figure 4. Result of Phase Spike
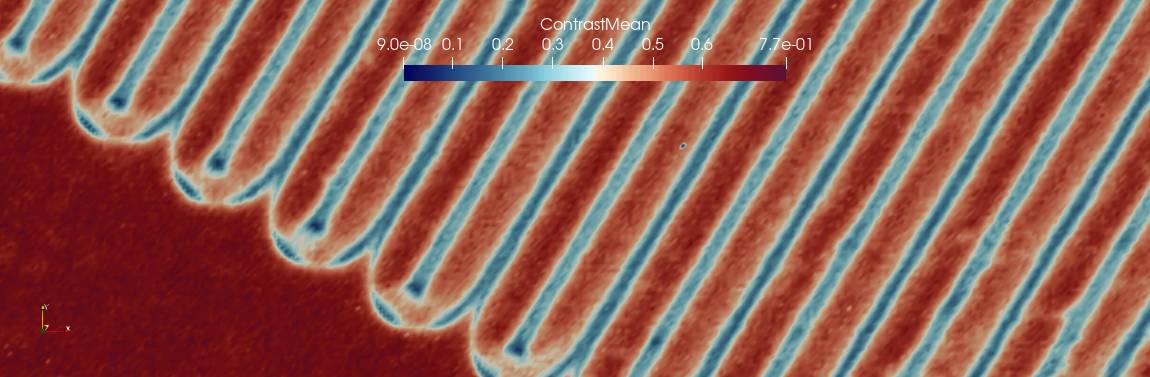
Figure 5. Overlapping errors : Low Contrast